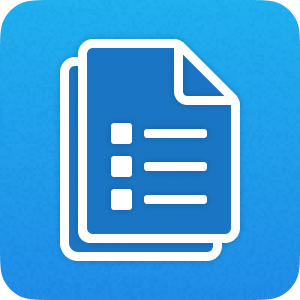
Multi-page Form
Quickly build a data service for a resumable multi-page form
Blueprints are a unique feature of Squiz Datastore (Datastore) and provide industry standard templates that enable rapid delivery of digital experiences on Squiz Matrix.
Frontend developers upload blueprints to the Squiz Experience Cloud to quickly build and manage API data services for website user interfaces.
Marketplace blueprints speed up the development process even further by providing onboarding examples and starting points for common use cases.
Use case
Build a form that has multiple pages/steps such as online wizards, online applications, surveys, and questionnaires. Capture data for a user, allow admins to see a list of all submissions, and remember the progress of users. Use this Datastore blueprint to automatically generate a data service that will enable the frontend to:
- Store unique documents per submission
- Store user name, email, and message
- Store and retrieve the page/step number a user is up to
- Retrieve data for unique documents e.g. form field pre-fill
- Allow admins to view a list of all submissions, protected by a JSON Web Token (JWT)
How to use
The following examples show how a JWT can be generated and tested in local development. JWT authentication in production can be implemented in conjunction with the Matrix JSON Web Token Extension where "isAdmin": true
must exist in the payload for the response to be successful.
1. Set up your local environment
Complete the Datastore event app tutorial to set up local development, understand core concepts, and if you need any support then please visit the Squiz Datastore Forums.
2. Simulate and test your blueprint
Assuming you are ready to use Datastore, then work through the following commands:
Download the blueprint:
curl -O https://marketplace.squiz.net/__data/assets/file/0016/36511/multi-page-form-blueprint.tgz
Unzip the the blueprint package:
tar -xvf multi-page-form-blueprint.tgz
Step into the blueprint directory:
cd multi-page-form-blueprint
Add the blueprint to your simulator, here is an example of what you might see:
dxp datastore simulator add --blueprint api.yaml
✔ Done! Use these details for local querying:
URL: http://ip.address:port/eK46TPXY
JWT URL: http://ip.address:port/__JWT/issueToken
Test with a simple curl command but replace the details highlighted in orange with details from the previous step. If successful, the same data will print to the terminal.
curl \
http://ip.address:port/eK46TPXY/submission \
-H "Content-Type: application/json" \
-X POST \
--data '{"name":"Jane","email":"jdoe@email.com","message":"Yay!", "currentPage":1}'
Now let's store a JWT for local testing of protected methods in your blueprint API. The method for storing an environment variable will vary, depending on your operating system. Run the following command in Linux systems:
export JWT_TOKEN=$(curl \
-s \
-X POST \
-H 'Accept: application/json' \
-H 'Content-Type: application/json' \
--data '{"isAdmin":true}' \
http://ip.address:port/__JWT/issueToken
);
View all protected form submissions using the locally stored token:
curl \
http://ip.address:port/eK46TPXY/submission \
-H "Content-Type: application/json" \
-H 'Authorization: Bearer '"${JWT_TOKEN}"''
If successful, you should see an array of document data, each with their own UUID called "submissionid".
3. Test and build using the JavaScript SDK
The following example code could be embedded in a script tag, or centrally managed inside of a main.js file.
First configure the Datastore JavaScript SDK in your app HTML for local testing:
<html>
....
<!-- use this sdk file for local simulation, remove this for production -->
<script src="https://sdk.datastore.squiz.cloud/js/sdk-simulator.js"></script>
<!-- swap to this file when you are ready for production -->
<!-- <script src="https://sdk.datastore.squiz.cloud/js/sdk.js"></script> -->
....
</html>
Now configure your settings, instantiate a new Datastore object, and simulate a JWT locally:
// JWT details are only used in local development
const settings = {
"serviceURL": "http://ip.address:port/eK46TPXY",
"jwtURL": "http://ip.address:port/__JWT/issueToken"
};
const datastore = new Datastore(settings);
datastore.setSetting('jwtPayload', {"isAdmin": true});
Setup your test data ready for querying:
let data = {
"name": "John",
"email": "jsmith@example.com",
"message": "My first JavaScript SDK test!",
"currentPage": 1
};
Create your first document with the test data:
datastore.collection('submission').add(data);
Print all document data to the console:
// You should see a promise printed to console with an array of data
console.log(datastore.collection('submission').get());
Print a submission by specific document ID:
let submissionid = "copy/paste one of the IDs printed in the previous step above";
console.log(datastore.collection('submission').doc(submissionid).get());
Complete the tutorial or review the reference guides for more JavaScript SDK methods.
4. Add your blueprint to the Squiz Experience Cloud
Assuming you have a login to the Squiz Experience Cloud and have activated a Datastore subscription, then login to the command line using your Squiz Experience Cloud email address, you will be prompted for your password:
dxp login --email emailaddress
Add your blueprint to production and give it a meaningful name:
dxp datastore blueprint add \
--path api.yaml \
--name "My Online Form"
List the details for your production blueprints:
dxp datastore blueprint list
Update an existing blueprint:
dxp datastore blueprint update \
--path boilerplate/api.yaml \
--name "My Online Form"
Rename an existing blueprint:
dxp datastore blueprint rename \
--old-name "My Online Form" \
--new-name "My Awesome Website App
5. Extend the fields captured
Extending the fields captured in the schema can be done by adding another property to the properties object in ./schemas/submission.json
, for example:
...
"properties": {
...
"newProperty": {
"title": "New Property",
"description": "Some new property",
"type": "boolean",
"example": false
}
}
...
Allowed JSON Schema property types:
- String (including format date, time and datetime)
- Number
- Integer
- Boolean
- Array (of type, mixed typed arrays are not allowed)
- null
The schema can also be extended to have required fields added. This is done by adding the required key to the JSON schema file, "required": ["message"]
this takes an array of property names from the properties object in the file.
Type | Blueprint |
---|---|
For | ![]() |
Documentation |