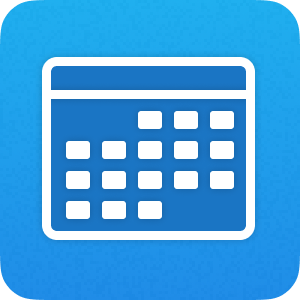
Book Meetings
Enable your customers to easily book meetings with your team, according to a schedule you set
Overview
Blueprints are a unique feature of Squiz Datastore (Datastore) and provide industry standard templates that enable rapid delivery of digital experiences on Squiz Matrix.
Frontend developers upload blueprints to the Squiz Experience Cloud to quickly build and manage API data services for website user interfaces.
Marketplace blueprints speed up the development process even further by providing onboarding examples and starting points for common use cases.
Implement this blueprint to enable your customers to easily book meetings with your team, according to a schedule you set.
When used in conjunction with the Squiz Connect recipe, both the customer and your staff member(s) will automatically receive a meeting invitation for the selected time, minimising overhead and human error.
With the addition of Squiz Datastore you can quickly and easily capture and surface the data you need to support your frontend user interfaces.
Implementation assumptions
The above scenario requires implementation with Squiz Matrix assets or a custom JavaScript application served by Squiz Matrix, or a mix of both. Implementation assumes:
- Admin users have to setup speakers and session information in Squiz Matrix: including the Squiz Matrix speaker profile and Squiz Matrix events for all available time slot. At the same time, the meeting records get dynamically added to Squiz Datastore.
- Site users visit the meeting sessions page and submit the booking form for a selected available meeting slot. The form submits to Squiz Connect and Squiz Connect checks the information including meeting host and selected meeting availability. If all information is valid, then Squiz Connect will create a meeting and update the Squiz Datastore meeting record (marked booked). If the information is invalid, Squiz Connect will respond with an error.
Installation
The following examples show how a JWT can be generated and tested in local development. JWT authentication in production can be implemented in conjunction with the Matrix JSON Web Token Extension where "isAdmin": true
must exist in the payload for the response to be successful.
1. Set up your local environment
Complete the Datastore event app tutorial to set up local development, understand core concepts, and if you need any support then please visit the Squiz Datastore Forums.
2. Simulate and test your blueprint
Assuming you are ready to use Datastore, then work through the following commands:
Download the blueprint:
curl -O https://marketplace.squiz.net/__data/assets/file/0026/36782/book-meetings-blueprint.tgz
Unzip the the blueprint package:
tar -xvf book-meetings-blueprint.tgz
Step into the blueprint directory:
cd book-meetings-blueprint
Add the blueprint to your simulator, here is an example of what you might see:
dxp datastore simulator add --blueprint api.yaml
✔ Done! Use these details for local querying:
URL: http://ip.address:port/eK46TPXY
JWT URL: http://ip.address:port/__JWT/issueToken
Test with a simple curl command but replace the details highlighted in orange with details from the previous step. If successful, the same data will print to the terminal.
curl \
http://ip.address:port/eK46TPXY/meeting-bookings \
-H "Content-Type: application/json" \
-X POST \
--data '{"meetingid":"1234","name":"John Smith","email":"jsmith@example.com"}'
Now let's store a JWT for local testing of protected methods in your blueprint API. The method for storing an environment variable will vary, depending on your operating system. Run the following command in Linux systems:
export JWT_TOKEN=$(curl \
-s \
-X POST \
-H 'Accept: application/json' \
-H 'Content-Type: application/json' \
--data '{"isAdmin":true}' \
http://ip.address:port/__JWT/issueToken
);
View all protected booking details using the locally stored token:
curl \
http://ip.address:port/eK46TPXY/meeting-bookings \
-H "Content-Type: application/json" \
-H 'Authorization: Bearer '"${JWT_TOKEN}"''
If successful, you should see an array of document data, each with their own UUID called "bookingid".
3. Test and build using the JavaScript SDK
The following example code could be embedded in a script tag, or centrally managed inside of a main.js file.
First configure the Datastore JavaScript SDK in your app HTML for local testing:
<html>
....
<!-- use this sdk file for local simulation, remove this for production -->
<script src="https://sdk.datastore.squiz.cloud/js/sdk-simulator.js"></script>
<!-- swap to this file when you are ready for production -->
<!-- <script src="https://sdk.datastore.squiz.cloud/js/sdk.js"></script> -->
....
</html>
Now configure your settings, instantiate a new Datastore object, and simulate a JWT locally:
// JWT details are only used in local development
const settings = {
"serviceURL": "http://ip.address:port/eK46TPXY",
"jwtURL": "http://ip.address:port/__JWT/issueToken"
};
const datastore = new Datastore(settings);
datastore.setSetting('jwtPayload', {"isAdmin": true});
Setup your test data ready for querying:
let data = {
"meetingid": "2345"
"name": "John",
"email": "jsmith@example.com"
};
Create your first document with the test data:
datastore.collection('meeting-bookings').add(data);
Print all document data to the console:
// You should see a promise printed to console with an array of data
console.log(datastore.collection('meeting-bookings').get());
Print a booking by specific document ID:
let bookingid = "copy/paste one of the IDs printed in the previous step above";
console.log(datastore.collection('meeting-bookings').doc(bookingid).get());
Complete the tutorial or review the reference guides for more JavaScript SDK methods.
4. Add your blueprint to the Squiz Experience Cloud
Assuming you have a login to the Squiz Experience Cloud and have activated a Datastore subscription, then login to the command line using your Squiz Experience Cloud email address, you will be prompted for your password:
dxp login --email emailaddress
Add your blueprint to production and give it a meaningful name:
dxp datastore blueprint add \
--path api.yaml \
--name "My Book Meetings App"
List the details for your production blueprints:
dxp datastore blueprint list
Update an existing blueprint:
dxp datastore blueprint update \
--path boilerplate/api.yaml \
--name "My Book Meetings App"
Rename an existing blueprint:
dxp datastore blueprint rename \
--old-name "My Book Meetings App" \
--new-name "My Awesome Website App
API Model
The book meetings blueprint provides two collections (meetings and meeting bookings) for developers to query document objects, with the following endpoints for Squiz Connect and developers to read and write data to:
Paths | Method | Security | Frontend Feature Mapping |
---|---|---|---|
/meetings | |||
GET | Publicly accessible. | Retrieve a list of all meetings. | |
/meetings/{meetingid} | |||
GET | Publicly accessible. | Lookup a meeting by ID. | |
PUT | "isAdmin": true | Add a meeting. | |
PATCH | "isAdmin": true | Update a meeting. | |
DELETE | "isAdmin": true | Delete a meeting. | |
/meeting-bookings | |||
GET | Publicly accessible. | Retrieve a list of all bookings. | |
POST | Publicly accessible. | Add a booking. | |
/meeting-bookings/{bookingid} | |||
GET | Publicly accessible. | Lookup a booking by ID. | |
DELETE | Publicly accessible. | Delete a booking. |
Data Model
Review the ./schemas/
folder for more details, here are the main objects and data types stored for this application.
Meeting booking summary
{
"$schema": "http://json-schema.org/draft-07/schema#",
"type": "object",
"properties": {
"bookingid": {
"x-datastore-source": "document.$id",
"description": "The ID of the booking."
},
"meetingid": {
"type": "string",
"description": "The meeting id for the booking"
}
}
}
Meeting booking
{
"$schema": "http://json-schema.org/draft-07/schema#",
"type": "object",
"properties": {
"meetingid": {
"x-datastore-source": "document.$id",
"description": "The ID of the meeting."
},
"title": {
"title": "Meeting title",
"description": "The name of the meeting.",
"type": "string"
},
"booked": {
"title": "Meeting status",
"description": "Status of the meeting.",
"type": "boolean",
"example": true
},
"created": {
"title": "Created date",
"description": "Meeting created date.",
"x-datastore-source": "document.$createdDatetime"
},
"updated": {
"title": "Updated date",
"description": "Meeting last updated date.",
"x-datastore-source": "document.$updatedDatetime"
}
},
"required": []
}
Meeting
{
"$schema": "http://json-schema.org/draft-07/schema#",
"type": "object",
"properties": {
"bookingid": {
"x-datastore-source": "document.$id",
"description": "The ID of the booking."
},
"meetingid": {
"type": "string",
"description": "The meeting id for the booking"
},
"name": {
"type": "string",
"example": "John Smith",
"description": "The name of the attendee."
},
"email": {
"type": "string",
"format": "email",
"example": "jsmith@example.com",
"description": "The email of the attendee."
},
"created": {
"x-datastore-source": "document.$createdDatetime",
"description": "The time the booking was made."
},
"updated": {
"x-datastore-source": "document.$updatedDatetime",
"description": "The time the booking was updated."
}
}
}
Extending the fields that are captured
Extending the fields captured in the schema can be done by adding another property to the properties objects in the ./schemas/
folder, for example:
...
"properties": {
...
"newProperty": {
"title": "New Property",
"description": "Some new property",
"type": "boolean",
"example": false
}
}
...
Allowed JSON Schema property types:
- String (including format date, time and datetime)
- Number
- Integer
- Boolean
- Array (of type, mixed typed arrays are not allowed)
- null
The schema can also be extended to have required fields added. This is done by adding the required key to the JSON schema file, "required": ["message"]
this takes an array of property names from the properties object in the file.
Type | Blueprint |
---|---|
For | ![]() |
Documentation |